Advent Calendar: Developing mobile apps at the speed of light.
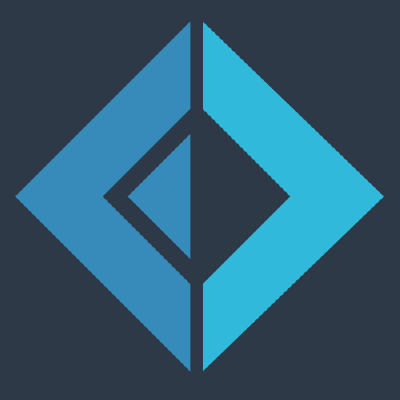
Have you heard about the FSharp TypeProviders? Type Providers allow you to accessto a defined data scheme with static typing, which means to have intellisense for your data source. You can create your own or use one that the community has already created. Of course, there is already a JSON Type Provider, it will allow you to consume a JSON based REST api in a statically typed way. This increases the productivity a lot, you don’t have to spend time anymore trying to figure out what the name of a field is (see video below). If you combine this with the conciseness of FSharp and the productivity in the View side thanks to Xamarin.Forms, you end up with a triple win. This makes FSharp the best choice for mobile development.
In this post I will try to show how to use the JSON Type Provider for building a Movies App using the TMDb API. There is an Apiary Type Provider too, but we won’t use it because the idea of this post is to figure out how to quickly build prototypes of your current APIs.
Creating the project
The first thing that we have to do is to create an iOS project for FSharp.
Then we have to install the Xamarin.Forms nugget package and also the FSharp.Data package which will contain the JSON Type Provider.
The next step is to add a few references from /Library/Frameworks/Mono.framework/Versions/Current/lib/mono/4.5/Facades/ you will need to System.ObjectModel.dll and System.Runtime.dll, System.Threading.Tasks, System.XML.ReaderWriter, System.Collections, System.Lin.Expressions.
Note that we could use a FSharp PCL Project for Xamarin.Forms (not sure if they are compatible with TypeProviders though). But I prefer to stay in the Xamarin Stable Channel because at the time of writing this, the FSharp PCL template is not in the Stable Channel.
I like to write clean models with the properties that I want to use, the types that best represent the domain, decoupled from the data source. For our example we will need just the Title, ImagePath and Overview of the film.
The TypeProvider Part
You will need to request a key for accessing to the API
The key thing is the JSONProvider. We have to pass a JSON sample (it can be an url, it will automatically download the JSON) and the provider will infer the types automatically. Notice that we have to mark the string as Literal, this means that the compiler will be able to access it. If we build now and we try to access an instance of the MovieList type, we will see that we have autocomplete! (behind the scenes the compiler auto generates the code for us).
We also defined a getPage function that will download the given page of movies. And another function, getMovies, that will allow us to download a bunch of pages at the same time. Notice that we won’t expose the types that the JSON Provider generates.
Making it work on iOS:
Notice that the sample should work on Android too. I will skip the Android part for keeping the post short, but the code shown above is CrossPlatform.
The view is pretty simple. If you are familiar with Xamarin.Forms, the code exposed here should be straightforward. Notice how easy it is to create an infinite scrolling with Xamarin.Forms and the function that we defined before.
And finally, our app delegate:
If you want to see the app in action, don’t forget to check out the video.
The code is here